In today’s world, wireless communication has become an integral part of our lives, and Bluetooth technology is one of the most widely used standards for short-range wireless communication. Whether you’re a hobbyist, a developer, or an engineer, understanding how to use Bluetooth on your board can open up a world of possibilities, from creating smart home devices to developing wearable technology.
This comprehensive guide will walk you through the process of setting up and using Bluetooth on your board, providing practical examples and code snippets to help you get started. We’ll cover everything from understanding the basics of Bluetooth technology to implementing real-world applications.
Understanding Bluetooth Technology
Before we dive into the practical aspects of using Bluetooth on your board, let’s briefly discuss what Bluetooth technology is and how it works.
What is Bluetooth?
Bluetooth is a wireless technology standard that enables short-range communication between devices. It operates in the 2.4 GHz radio frequency (RF) band and uses frequency-hopping spread spectrum (FHSS) technology to reduce interference and provide secure data transmission.
Bluetooth Versions and Specifications
Over the years, Bluetooth has evolved through several versions, each introducing new features and improvements. Here’s a brief overview of the most recent versions:
Version | Release Year | Key Features |
Bluetooth 5.2 | 2020 | Higher data transfer rates, improved security, and better energy efficiency |
Bluetooth 5.1 | 2019 | Improved location services and better support for IoT devices |
Bluetooth 5.0 | 2016 | Increased range, higher data transfer rates, and improved energy efficiency |
Bluetooth 4.2 | 2014 | Better privacy, higher data transfer rates, and improved power consumption |
The latest versions of Bluetooth offer better performance, security, and energy efficiency, making them well-suited for a wide range of applications, from low-power IoT devices to high-speed data transfers.
Setting up Bluetooth on Your Board
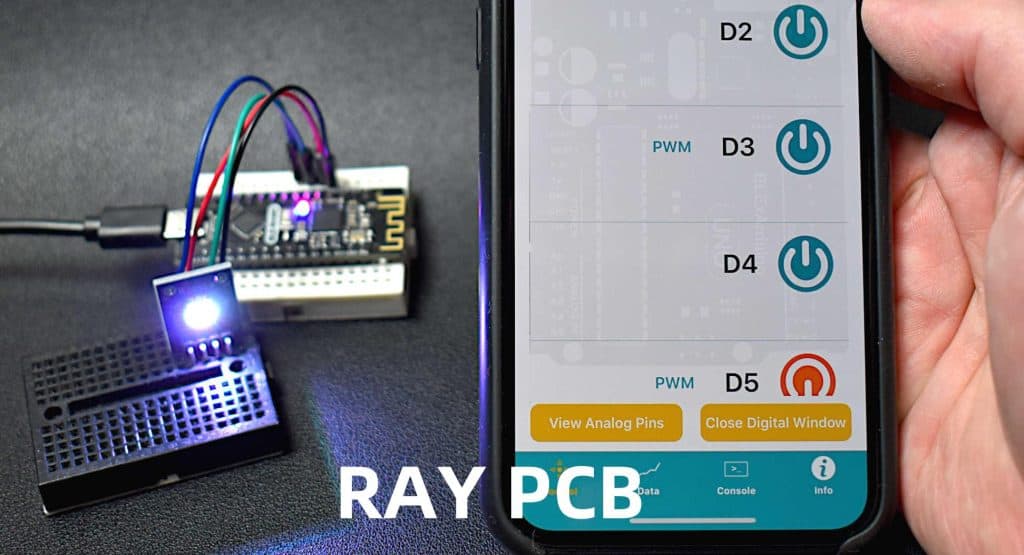
Before you can start using Bluetooth on your board, you’ll need to set it up properly. The setup process may vary depending on the specific board you’re using, but here are some general steps to follow:
- Choose a Bluetooth Module: Select a Bluetooth module that is compatible with your board. Popular options include the HC-05, HC-06, and HM-10 modules, which are widely available and relatively inexpensive.
- Connect the Module: Connect the Bluetooth module to your board according to the pinout and wiring instructions provided by the manufacturer.
- Install Libraries and Dependencies: Depending on your board and the programming language you’re using, you may need to install specific libraries or dependencies to enable Bluetooth communication. For example, if you’re using an Arduino board with the Arduino IDE, you’ll need to install the SoftwareSerial or AltSoftSerial library.
- Configure the Bluetooth Module: Most Bluetooth modules require some initial configuration, such as setting the baud rate, pairing mode, and device name. You can typically do this using a serial terminal or a dedicated configuration tool provided by the manufacturer.
Once you’ve completed these steps, your board should be ready to communicate with other Bluetooth-enabled devices.
RayMing PCB HM-20 cc2340 BLE5.3 Bluetooth Module Transmission Compatible with Multi-point Links
Master-Slave Integration Remote Control Firmware Upgrade Command Rich
Practical Examples
Now that you have a basic understanding of Bluetooth technology and have set up your board, let’s dive into some practical examples of how to use Bluetooth on your board.
Example 1: Bluetooth Serial Communication
One of the most common use cases for Bluetooth on a board is to establish serial communication between two devices. This can be useful for various applications, such as wirelessly transmitting sensor data, controlling devices remotely, or exchanging messages between devices.
Here’s an example of how you can establish a Bluetooth serial connection between an Arduino board and a computer using the SoftwareSerial library:
arduinoCopy code// Include the SoftwareSerial library
#include <SoftwareSerial.h>
// Define the Bluetooth module pins
#define RX_PIN 2
#define TX_PIN 3
// Create a SoftwareSerial object
SoftwareSerial bluetooth(RX_PIN, TX_PIN);
void setup() {
// Initialize the serial communication
Serial.begin(9600);
bluetooth.begin(9600);
// Print a message to indicate the start of the program
Serial.println("Bluetooth Serial Communication Example");
}
void loop() {
// Check if data is available from the Bluetooth module
if (bluetooth.available()) {
// Read the incoming data and print it to the serial monitor
char data = bluetooth.read();
Serial.print(data);
}
// Check if data is available from the serial monitor
if (Serial.available()) {
// Read the incoming data and send it to the Bluetooth module
char data = Serial.read();
bluetooth.print(data);
}
}
In this example, we create a SoftwareSerial
object to communicate with the Bluetooth module. The setup()
function initializes the serial communication with the computer and the Bluetooth module at a baud rate of 9600. The loop()
function continuously checks for incoming data from both the Bluetooth module and the serial monitor, and echoes the received data back to the other side.
To test this example, you can open the serial monitor in the Arduino IDE and send messages to the board. Any messages sent from the serial monitor will be transmitted to the Bluetooth module, and vice versa.
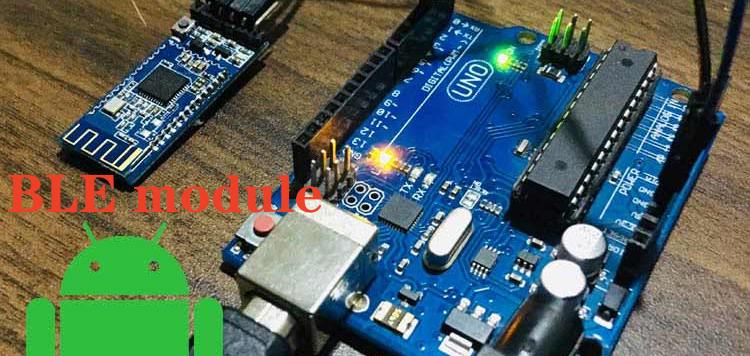
Example 2: Bluetooth Remote Control
Another practical application of Bluetooth on your board is to create a remote control system. This can be useful for controlling various devices, such as robots, home automation systems, or even multimedia players.
Here’s an example of how you can create a simple Bluetooth remote control system using an Arduino board and a mobile app:
arduinoCopy code// Include the SoftwareSerial library
#include <SoftwareSerial.h>
// Define the Bluetooth module pins
#define RX_PIN 2
#define TX_PIN 3
// Define the LED pin
#define LED_PIN 13
// Create a SoftwareSerial object
SoftwareSerial bluetooth(RX_PIN, TX_PIN);
void setup() {
// Initialize the serial communication
Serial.begin(9600);
bluetooth.begin(9600);
// Set the LED pin as an output
pinMode(LED_PIN, OUTPUT);
// Print a message to indicate the start of the program
Serial.println("Bluetooth Remote Control Example");
}
void loop() {
// Check if data is available from the Bluetooth module
if (bluetooth.available()) {
// Read the incoming data
char data = bluetooth.read();
// Check if the received data is '1' or '0'
if (data == '1') {
// Turn on the LED
digitalWrite(LED_PIN, HIGH);
Serial.println("LED ON");
} else if (data == '0') {
// Turn off the LED
digitalWrite(LED_PIN, LOW);
Serial.println("LED OFF");
}
}
}
In this example, we use the same setup as in the previous example, but we add an LED to the board to demonstrate the remote control functionality. The loop()
function checks for incoming data from the Bluetooth module, and if the received data is ‘1’, it turns the LED on, and if the received data is ‘0’, it turns the LED off.
To control the LED remotely, you can use a Bluetooth-enabled mobile app that can send the ‘1’ and ‘0’ characters to the board. There are various mobile apps available for different platforms (e.g., Android, iOS) that can be used for this purpose.
Example 3: Bluetooth Data Logging
Another practical application of Bluetooth on your board is to create a data logging system. This can be useful for collecting and storing sensor data, environmental data, or any other type of data that needs to be transmitted wirelessly.
Here’s an example of how you can create a simple Bluetooth data logging system using an Arduino board:
arduinoCopy code// Include the SoftwareSerial library
#include <SoftwareSerial.h>
// Define the Bluetooth module pins
#define RX_PIN 2
#define TX_PIN 3
// Define the sensor pin
#define SENSOR_PIN A0
// Create a SoftwareSerial object
SoftwareSerial bluetooth(RX_PIN, TX_PIN);
void setup() {
// Initialize the serial communication
Serial.begin(9600);
bluetooth.begin(9600);
// Print a message to indicate the start of the program
Serial.println("Bluetooth Data Logging Example");
}
void loop() {
// Read the sensor value
int sensorValue = analogRead(SENSOR_PIN);